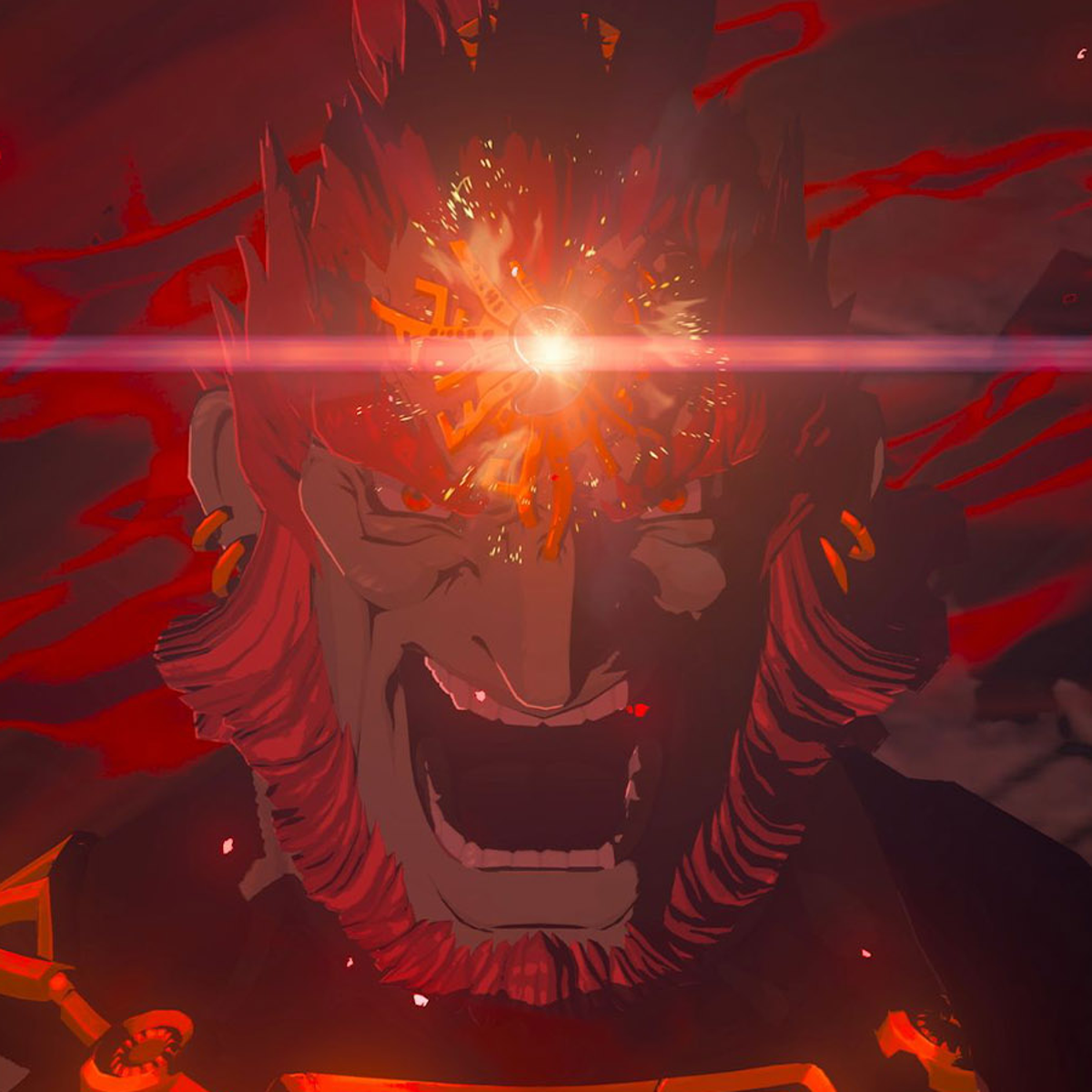
Ganondorf @ganon_dorf · 2024-07-03
Planning my next move...
Ganondorf @ganon_dorf · 2024-07-03
Planning my next move...
Zelda @zelda · 2024-07-02
Defeated Ganon and restored peace to Hyrule.
Mipha @mipha · 2024-07-09
Healing my friends with Mipha's Grace.
Urbosa @urbosa · 2024-07-08
Training hard to master Urbosa's Fury.
Link @link_hero · 2024-07-01
Just found the Master Sword in the Lost Woods!
Impa @impa · 2024-07-10
Researching ancient Sheikah technology.
Prince Sidon @sidon · 2024-07-05
Helped Link defeat the Divine Beast Vah Ruta.
Daruk @daruk · 2024-07-07
Protected my friends with Daruk's Protection today.
Revali @revali_the_great · 2024-07-06
My flight skills are unmatched. Watch out, Ganon!
Beedle @buy_from_beedle · 2024-07-04
Come buy from Beedle! Best deals in Hyrule!
useMemo
?useMemo
is a hook that allows you to cache the result of a calculation between re-renders
Memoization is an optimization technique used to speed up calculations by storing the result of expensive function calls and returning the cached result when the same inputs occur again.
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
Optimizing performance for heavy calculations (like filtering / sorting large arrays)
Improving performance in large data tables
useMemo
Expected Logs:
<Feed />
component rendergetSortedPosts
renderconst getSortedPosts = (feedPosts, sort) => {
console.log(`[NOT MEMO] running sort by ${sort}`);
switch (sort) {
case "Most Popular":
return [...feedPosts].sort((a, b) => b.likes - a.likes);
case "Most Comments":
return [...feedPosts].sort((a, b) => b.comments - a.comments);
case "Most Recent":
default:
return [...feedPosts].sort(
(a, b) =>
new Date(b.datePosted).getTime() - new Date(a.datePosted).getTime(),
);
}
};
<hr />;
const sortedPosts = getSortedPosts(feedPosts, sort);
useMemo
Expected Logs:
<Feed />
component rendergetSortedPosts
renderuseMemo
Expected Logs:
<Feed />
component rendergetSortedPosts
render (on initial render, not forced update)const sortedPosts = useMemo(() => {
console.log(`[WITH MEMO] running sort by ${sort}`);
switch (sort) {
case "Most Popular":
return [...feedPosts].sort((a, b) => b.likes - a.likes);
case "Most Comments":
return [...feedPosts].sort((a, b) => b.comments - a.comments);
break;
case "Most Recent":
default:
return [...feedPosts].sort(
(a, b) =>
new Date(b.datePosted).getTime() - new Date(a.datePosted).getTime(),
);
}
}, [feedPosts, sort]);
useMemo
Expected Logs:
<Feed />
component rendergetSortedPosts
render (on initial render, not forced update)