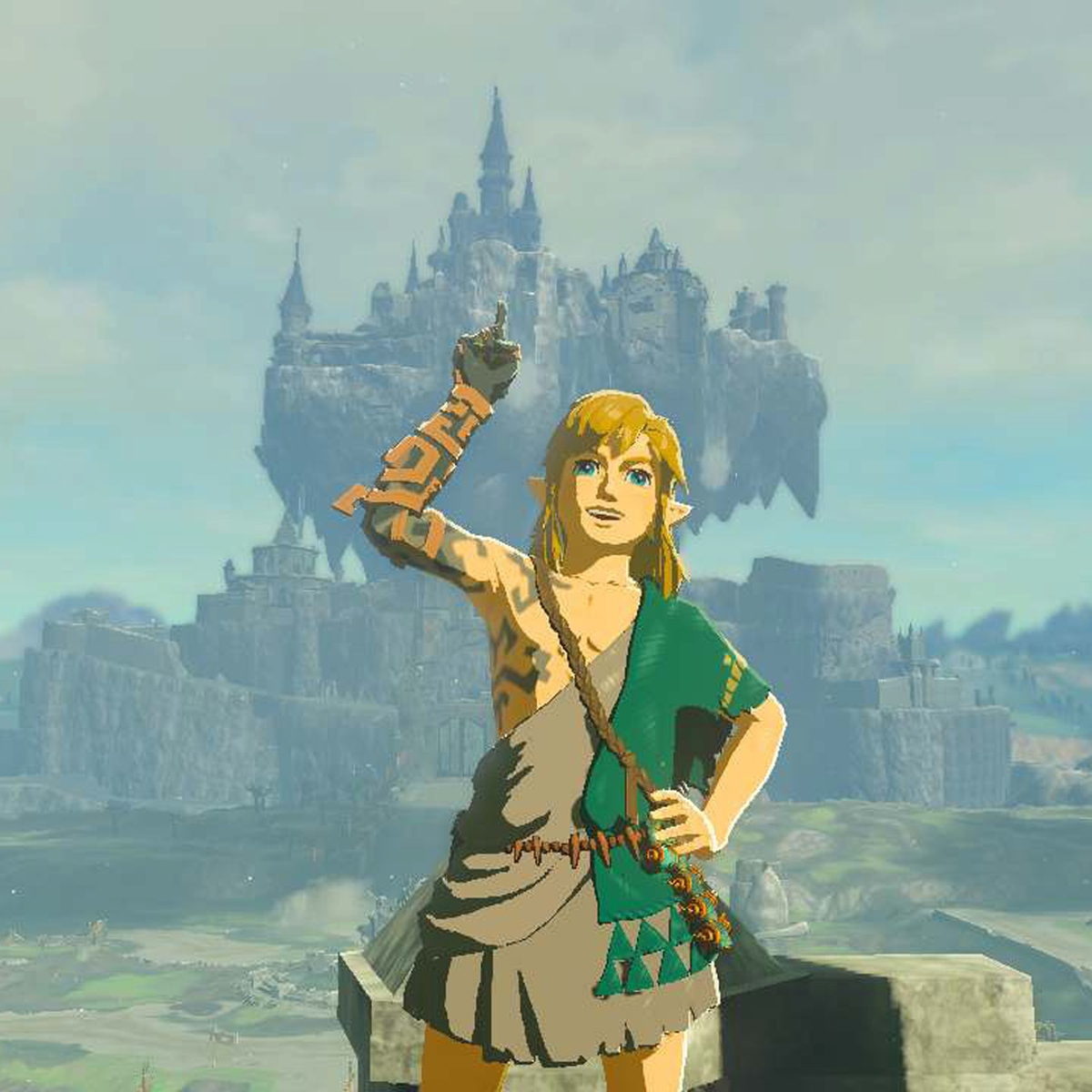
Link @link Β· 2m
Just found another Korok seed! These little guys are everywhere! π± #HyruleAdventures #KorokHunt
@link
π Adventuring across realms to restore peace and balance. β’ π£ Not much of a talker
Hyrule
Link @link Β· 2m
Just found another Korok seed! These little guys are everywhere! π± #HyruleAdventures #KorokHunt
Link @link Β· 1h
Cooking up some mighty meals today. Anyone got a good recipe for Dubious Food? π² #CookingWithLink
Link @link Β· 5h
Stumbled upon an ancient shrine. The puzzles in these never get old! π§© #ShrineSeeker #ZeldaPuzzleMaster
Link @link Β· 2d
Finally upgraded my armor! Feeling invincible now. πͺ #ArmorUpgrade #HyruleWarrior
Link @link Β· 07/22/2024
Defeated Ganon again. When will he learn? #HeroOfHyrule #NeverGiveUp
useState
?useState
is the hook that allows you to manage local state within your React components
const [count, setCount] = useState(0);
Simple state management where the state is independent and doesnβt involve complex logic or multiple values that need to be updated together.
Examples:
useState
// TriforceTap.tsx
import React, { useState } from "react";
const TriforceTap = () => {
const [totalTriforceTaps, setTotalTriforceTaps] = useState(0);
const [allowSelfTap, setAllowSelfTap] = useState(true);
const handleTap = () => {
setTotalTriforceTaps(totalTriforceTaps + 1);
setAllowSelfTap(false);
};
return (
<div>
<p>{totalTriforceTaps}</p>
<button onClick={handleTap} disabled={!allowSelfTap}>
{" "}
Tap{" "}
</button>
</div>
);
};
// TriforceTap.tsx
import React, { useState } from "react";
const TriforceTap = () => {
const [totalTriforceTaps, setTotalTriforceTaps] = useState(0);
const [allowSelfTap, setAllowSelfTap] = useState(true);
const handleTap = () => {
setTotalTriforceTaps((prevTaps) => prevTaps + 1); // ππΎ
setAllowSelfTap(false);
};
return (
<div>
<p>{totalTriforceTaps}</p>
<button onClick={handleTap} disabled={!allowSelfTap}>
Tap
</button>
</div>
);
};
// TriforceTap.tsx
// β Instead of having three pieces of state
const [totalTriforceTaps, setTotalTriforceTaps] = useState(0);
const [allowSelfTap, setAllowSelfTap] = useState(true);
const [coolDown, setCoolDown] = useState(0);
// β
Create one state object for multiple pieces of related state
const coolDownPeriod = 5; //in seconds
const [state, setState] = useState({
totalTriforceTaps: 0,
allowSelfTap: true,
coolDown: 0,
});
// TriforceTap.tsx
const coolDownPeriod = 5; //in seconds
// Updating the state when the tap button is clicked
const handleTap = () => {
setState((prevState) => ({
...prevState,
totalTriforceTaps: prevState.totalTriforceTaps + 1,
allowSelfTap: false,
coolDown: coolDownPeriod,
}));
};
useEffect
to create a cool down timer// Defines the count down timer and updates the button state when the cool down period is over
useEffect(() => {
let timer;
if (state.coolDown > 0) {
timer = setInterval(() => {
setState((prevState) => ({
...prevState,
coolDown: prevState.coolDown - 1,
allowSelfTap: prevState.coolDown - 1 <= 0,
}));
}, 1000);
}
return () => clearInterval(timer);
}, [state.coolDown]);
useState
if: